Why I Write Unit Tests
In computer programming, unit testing is a software testing method by which individual units of source code, sets of one or more computer program modules together with associated control data, usage procedures, and operating procedures, are tested to determine whether they are fit for use — wikipedia
Unit tests, who needs em? We all do. There are a lot of resources online that will tell you why unit testing is important or why writing unit tests before building implementations is a good idea. But I’m here to tell you how unit tests can save you a massive production headache.
A while back, a coworker pushed code to production that consumed and parsed large .csv files uploaded by users. The code looped through the file then parsed and mapped each record to the associated record in the model. The model contained a few bool fields that were populated by executing the C# Boolean.TryParse method against the text field to convert the string from the file to bool. If the TryParse method returned false then the value in the csv file must have been falsy, and vice versa for true. The record was applied to the model and saved to the database.
As it turned out, our code didn’t work as expected which caused a major problem. For bool fields users would upload either a 1 or 0. 1 being true and 0 being false. Because the source was a csv file all values were imported as strings. Therefore, before applying the values to our model, we must convert the string representation to the appropriate type within the model.
Here’s an example of the code, the Parse method below takes a string argument named parsable, does a Boolean.TryParse against parsable and returns the newly converted value.
public static Main(){ var trueFalse = Parse("1"); } public bool Parse(string parsable) { bool flag; bool result = Boolean.TryParse(parsable, out flag); return flag; }
From looking at the method and without knowing how C# Boolean.TryParse converts the string argument “1” to bool you’d think that this method returns true, not false. But in reality the above method returns false. Even though 1 generally equals true in most contexts, if passed in as a string the result is false, not true.
If we would have had proper unit tests set up we would have written a unit test that looks something like this:
[TestMethod]public void Parse_BooleanTryParseString__ReturnsFalse(){ string stringToParse = "1"; bool expected = true; bool actual = Parse(stringToParse); Assert.AreEqual(expected, actual);}
The above unit test would have failed and we’d have found the problem, fixed it and been on our way. Unfortunately for us, we didn’t have a unit test for the above Parse method and QA didn’t catch the issue during their testing. The code eventually made its way to production, users start uploading files against the new code and yadda, yadda, yadda…we end up with a lot false values that should be true.
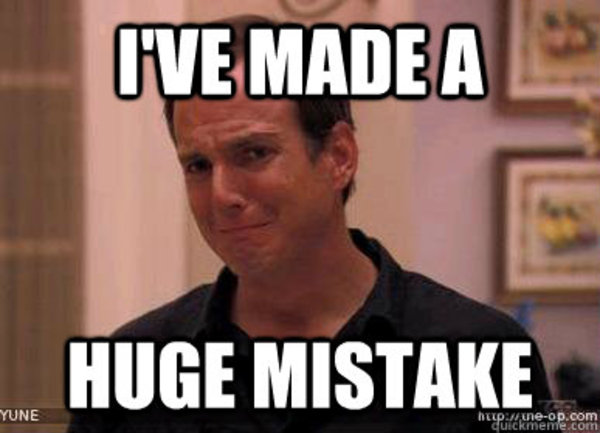
The good news is we were able to recover the lost data. But the recovery process took a few days and a lot of manual work which set us back on our timeline, not to mention created a garbage truck full of unwanted stress while we scrambled to recover the data and explain to our stakeholders that we’d made a huge mistake.
The takeaway my team and I had from this experience is how valuable a unit test can be. Unit tests are not just a key part of the testing process, but they’ve proven to be invaluable in making sure the code you write is actually doing what you think it’s doing.